This code defines a graphical user interface (GUI) application for filtering and displaying financial data. Here's a breakdown of the functionality:
Imports:
tkinter: Provides the core widgets for building the GUI.
ttk: Offers additional widgets with a more modern look and feel.
messagebox: Used for displaying pop-up messages (info and error).
json: Enables working with JSON formatted data (financial information).
os: Used for file path manipulation.
Functions:
load_json_file(file_path): Opens a JSON file and returns the loaded data.
filter_companies(companies_data, filters... ): Takes a list of companies and various filter criteria (city, sector, index, ticker), then returns a list of companies that match the filters.
select_preferred_json_file(ticker, year, period, base_directory): Based on the provided ticker, year, period, and directory path, searches for a preferred JSON file format and returns its path if found. Prints debugging information during the search process.
display_financial_tables(json_data): Takes JSON data (assumed to contain financial tables) and tries to extract and display the "Balance Sheet", "Income Statement", and "Cash Flow" sections. If not found directly under these names, it looks for them under a "tables" key. Returns a formatted string containing the extracted financial tables or a message indicating no tables were found.
Class FinancialDataApp:
This class represents the main application window.
init(self, root): The constructor initializes the root window (root) and sets the window title.
create_widgets(self): Creates all the GUI elements within the window:
Dropdown menus for filtering by city, sector, index, and ticker code.
Entry fields (disabled) for year and period (presumably pre-defined values).
Entry fields for including and excluding item filters (comma-separated).
A button to process data based on the selected filters.
A text area to display the results (company names, tickers, and financial tables).
load_dropdown_data(self): Loads initial data for the dropdown menus (cities, sectors, indices, and tickers) from specified file paths.
update_ticker_dropdown(self, event): Handles user input in the ticker code dropdown. As the user types, the list is filtered to show only options containing the entered characters. Similar update functions are defined for the other dropdown menus (city, sector, and index).
process_data(self): This function is triggered when the "Load and Process" button is clicked. It:
Retrieves user input from the various input fields.
Loads all company data from a JSON file.
Applies filtering based on the selected criteria (city, sector, index, and ticker code).
Iterates through the filtered companies:
Displays company name and tickers.
Calls select_preferred_json_file to find the relevant JSON file for each ticker.
If a JSON file is found, it calls display_financial_tables to extract and show the financial tables.
If no JSON file is found, it displays a message indicating that.
In case of any errors during processing, it displays an error message box.
Overall, this code provides a user-friendly interface for filtering companies based on various criteria and then displaying their corresponding financial data from JSON files.Metni buraya yazın...
This Python code creates a GUI application for filtering and displaying financial data. It allows users to select filters for ticker codes, cities, sectors, and indices, and then displays relevant financial data from JSON files.
Code info;
Total length: 285 lines
13756 chars
24.08.2024 - Build date.
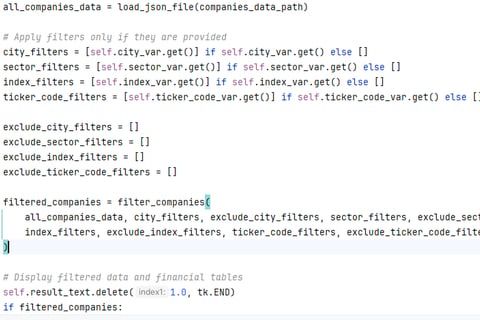
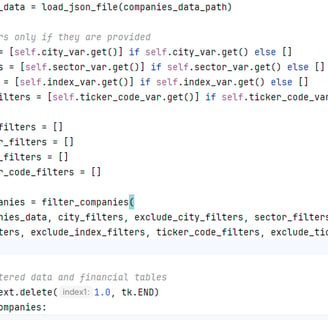
More details
The non GUI version of this code has 141 lines of code.
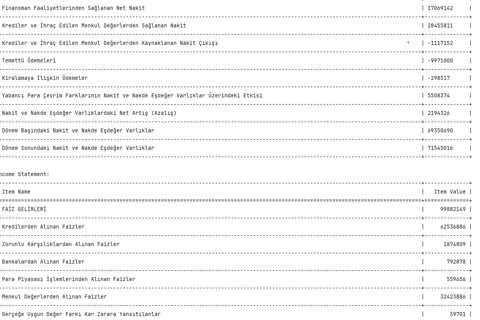
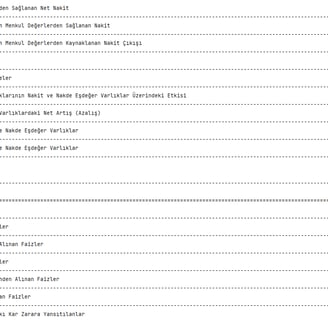